Basic Concepts in Python
Lesson Three Basic Concepts: Variables, Data Types, Arithmetic Operations, and Conditional Structures
Learn Python
February 1, 2025
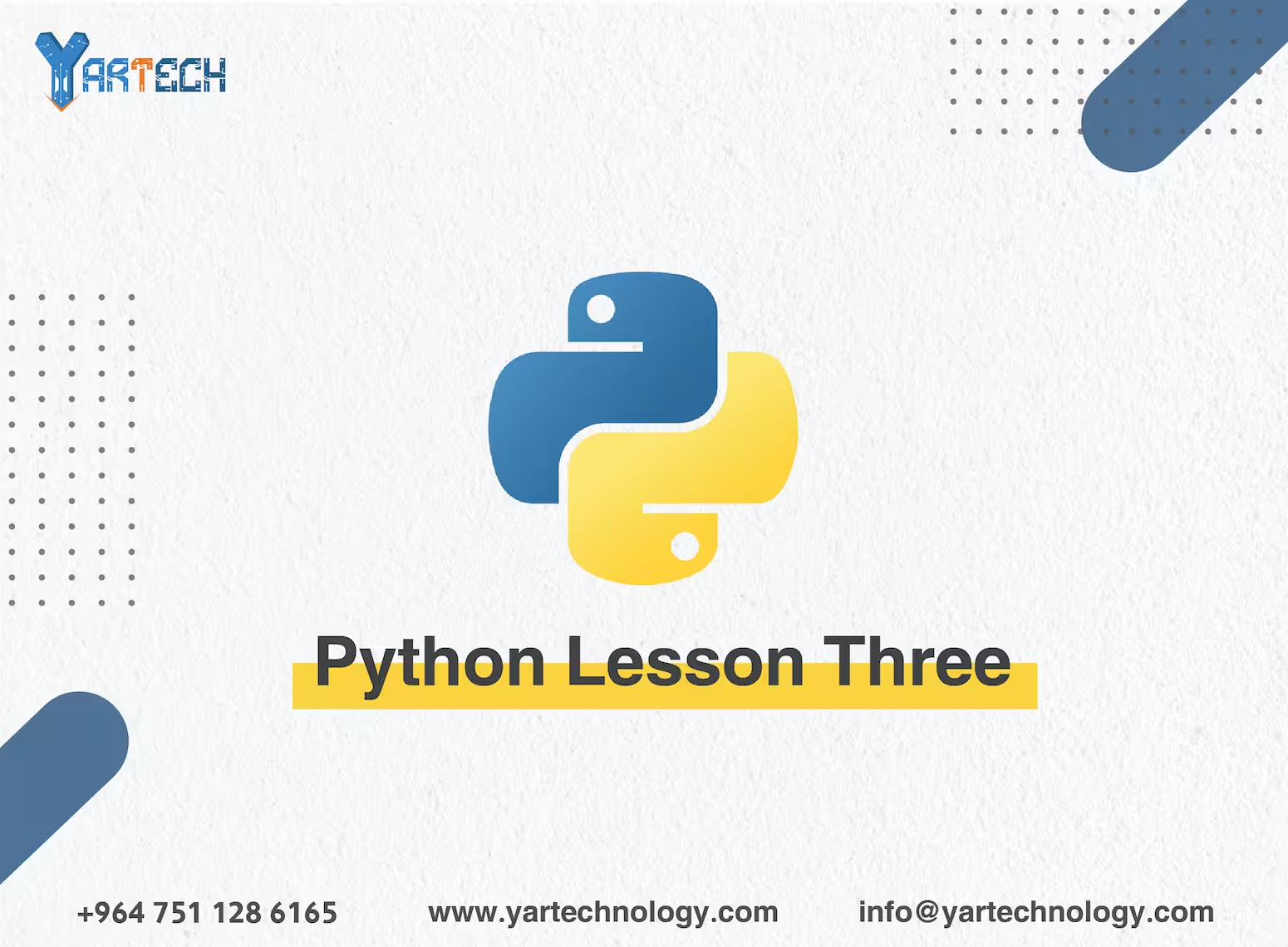
Lesson Three: Basic Concepts in Python
Welcome to the third lesson in our Python learning series! In this lesson, we will review the fundamental concepts you need to understand well in order to start writing useful programs. We will discuss variables, data types, arithmetic operations, and conditional structures. These concepts are the foundation upon which everything you will learn later will be built.
1. Variables
Variables are storage tools used to hold data that can be used later in the program. In Python, you don't need to explicitly specify the type of the variable; the language automatically identifies it based on the value stored in it.
How to Define Variables
x = 10 # Integer
y = 3.14 # Float
name = "Ali" # String
is_active = True # Boolean
Variable Naming Rules
- Must start with a letter or an underscore (_).
- Cannot start with a number.
- Cannot contain spaces, but you can use underscores (_) between words.
- Cannot be reserved keywords like
if
,else
,for
.
Examples:
my_variable = 5
_myVariable = 10
myVariableName = "Python"
2. Data Types
Python supports many data types, each with its own specific uses. The basic types are:
A. Numbers
- Integers (int): Such as
1
,-5
,100
. - Floats (float): Such as
3.14
,-0.001
,2.0
.
age = 25 # Integer
height = 1.75 # Float
B. Strings
A string is a sequence of characters or symbols, written within single quotes ' '
or double quotes " "
.
name = "Ahmed"
greeting = 'Hello, World!'
C. Booleans
Boolean values are either True
or False
. They are often used in conditional operations.
is_student = True
is_adult = False
D. Lists
Lists are ordered collections of items and can contain different types of data.
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4]
mixed = [1, "apple", 3.14, True]
E. Dictionaries
Dictionaries are collections of key-value pairs.
person = {
"name": "Ali",
"age": 25,
"is_student": True
}
3. Arithmetic Operations
Python supports all basic arithmetic operations such as addition, subtraction, multiplication, and division. Here are some examples:
A. Basic Operations
a = 10
b = 3
print(a + b) # Addition: 13
print(a - b) # Subtraction: 7
print(a * b) # Multiplication: 30
print(a / b) # Division: 3.333...
print(a % b) # Modulus (remainder): 1
print(a ** b) # Exponentiation: 1000 (10^3)
B. Operator Precedence
Python follows mathematical precedence rules (PEMDAS):
- Parentheses
()
- Exponents
**
- Multiplication and Division
*
and/
- Addition and Subtraction
+
and-
result = 10 + 3 * 2 # Result: 16 (Multiplication comes before addition)
result = (10 + 3) * 2 # Result: 26 (Parentheses take precedence)
4. Conditional Structures
Conditional structures are used to make decisions inside the program based on certain conditions. The main keywords used are if
, elif
, and else
.
A. Basic Structure
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
B. Logical Conditions
You can use logical operators like and
, or
, and not
to combine multiple conditions.
age = 20
is_student = True
if age > 18 and is_student:
print("You are an adult student.")
elif age > 18 or is_student:
print("You are an adult or a student.")
else:
print("You are still young.")
5. Loops
Loops are used to repeat the execution of a piece of code multiple times. There are two main types of loops in Python: for
and while
.
A. for
Loop
Used to repeat code for a specific number of times or to iterate over elements in a list.
# Print numbers from 1 to 5
for i in range(1, 6):
print(i)
# Iterate through a list
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
B. while
Loop
Used to repeat code as long as the condition is true.
count = 1
while count <= 5:
print(count)
count += 1
6. Functions
Functions are blocks of code that perform a specific task and can be called whenever needed. Functions are used to organize and reuse code.
A. Defining a Function
def greet(name):
print(f"Hello {name}!")
greet("Ali") # Output: Hello Ali!
B. Function Returning a Value
def add(a, b):
return a + b
result = add(5, 3)
print(result) # Output: 8
Practical Applications
Application 1: Simple Calculator
def calculator():
num1 = float(input("Enter the first number: "))
operator = input("Enter the operation (+, -, *, /): ")
num2 = float(input("Enter the second number: "))
if operator == "+":
print(num1 + num2)
elif operator == "-":
print(num1 - num2)
elif operator == "*":
print(num1 * num2)
elif operator == "/":
print(num1 / num2)
else:
print("Invalid operation.")
calculator()
Application 2: Age Verification
def check_age(age):
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
check_age(20) # Output: You are an adult.
check_age(15) # Output: You are a minor.
Conclusion
In this lesson, you learned the basic concepts you will need to write programs using Python. These concepts include variables, data types, arithmetic operations, conditional structures, and loops. These fundamentals will enable you to start building more complex programs.
In the next lesson, we will explore more advanced concepts such as lists, dictionaries, and file handling. Keep practicing and don't hesitate to ask questions if you need help!
🚀 Keep learning and practicing—you're on the right path to becoming a professional programmer!
Comments
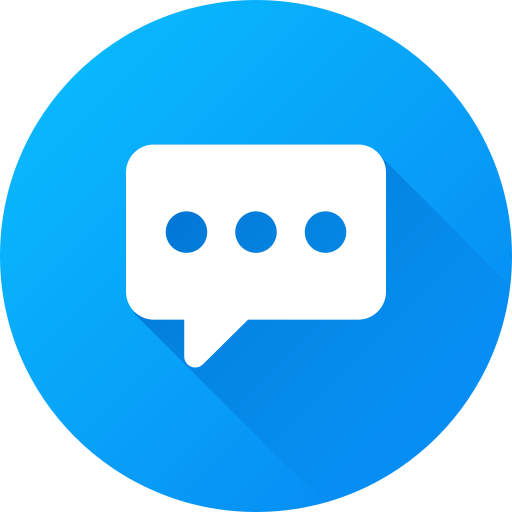
There are no comments
Please login to leave a review