Modules in Python
Lesson Eight of Python: Modules and how to use them to reuse and organize code better.
Learn Python
February 5, 2025
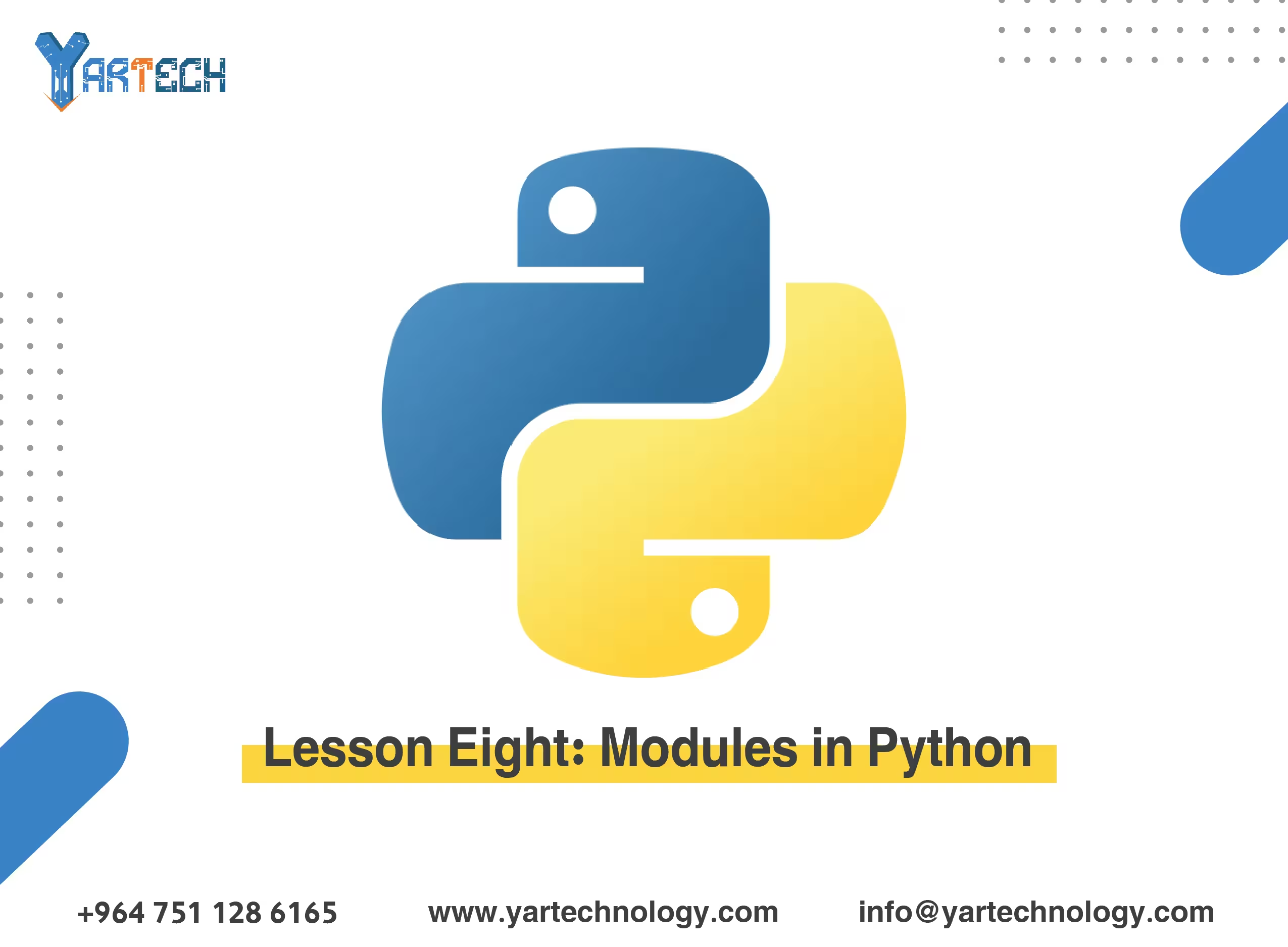
Lesson Eight: Modules in Python
Welcome to the eighth lesson in our Python learning series! In this lesson, we will focus on modules and how to use them to reuse and better organize your code. Modules are powerful tools that help you break down a program into smaller, manageable parts, making your code more organized and reusable.
1. What Are Modules?
A module is a file containing Python code, such as functions, classes, or variables. Modules can be written by you, part of Python's standard libraries, or even external libraries installed using the package manager pip
.
Examples of Standard Modules in Python
- math : A module that contains mathematical functions.
- random : A module used to generate random numbers.
- os : A module for interacting with the operating system.
- datetime : A module for working with dates and times.
2. How to Import Modules
To access the functionality of a module in your program, you can use the import
keyword. There are several ways to import modules:
A. Importing the Entire Module
B. Importing a Module with an Alias
If you want to shorten the module name, you can use the as
keyword.
import math as m
print(m.sqrt(16)) # Output: 4.0
C. Importing Specific Functions or Variables from a Module
If you only need a specific function or variable from a module, you can import it directly.
D. Importing Everything from a Module
You can import all functions and variables from a module using *
.
Note : Although using *
makes it easier to access all functions, it may lead to naming conflicts if there are functions with the same name in different modules. Therefore, it's better to import only the functions you need.
3. Creating Your Own Modules
You can also create your own modules. A module is simply a Python file containing functions or variables. Let's create a simple module.
A. Creating the Module
Suppose you have a file named mymodule.py
containing the following functions:
# mymodule.py
def greet(name):
print(f"Hello, {name}!")
def add(a, b):
return a + b
B. Using the Module in Another Program
You can now import this module and use the functions defined in it.
4. Common Standard Modules
A. math
Module
Used for performing mathematical operations.
import math
print(math.pi) # Output: 3.141592653589793
print(math.sqrt(16)) # Output: 4.0
print(math.factorial(5)) # Output: 120
B. random
Module
Used for generating random numbers.
C. os
Module
Used for interacting with the operating system, such as creating files or reading paths.
import os
print(os.getcwd())
# Get the current working directory
os.mkdir("new_folder")
# Create a new folder
D. datetime
Module
Used for working with dates and times.
5. Installing External Modules Using pip
In addition to standard modules, there are many external libraries that you can use. These libraries are installed using the package manager pip
.
A. Installing an External Library
For example, to install the requests
library, which is used for handling HTTP requests:
pip install requests
B. Using the Library After Installation
6. Practical Applications
Application 1: Using the math
Module
import math
radius = 5
area = math.pi * math.pow(radius, 2)
print(f"The area of the circle is: {area}")
# Output: The area of the circle is: 78.53981633974483
Application 2: Using the random
Module
Application 3: Using the datetime
Module
7. Tips for Using Modules
-
Use Standard Modules First :
Before looking for external libraries, try using the standard modules because they are built into Python and don't require installation. -
Organize Code Using Modules :
If your program is large, try dividing it into smaller modules for each part of the program. -
Avoid Importing Everything Using
*
:
As mentioned earlier, importing everything may lead to naming conflicts. Try importing only the functions or variables you need. -
Use
pip
Carefully :
When installing external libraries, make sure they are reliable and up-to-date.
Conclusion
In this lesson, you learned how to use modules in Python to reuse and better organize your code. Modules are powerful tools that help you break down a program into smaller, manageable parts.
In the next lesson, we will discuss Object-Oriented Programming (OOP) and how to use classes and objects in Python. Keep practicing and don't hesitate to ask questions if you need help!
🚀 You're now on the right path to becoming a professional programmer!
Comments
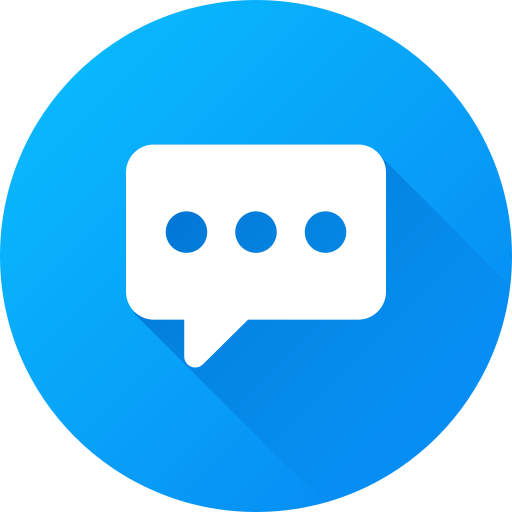
There are no comments
Please login to leave a review