Object-Oriented Programming (OOP)
Lesson Nine of Python: Object-Oriented Programming (OOP), a programming style that relies on the use of classes and objects.
Learn Python
February 5, 2025
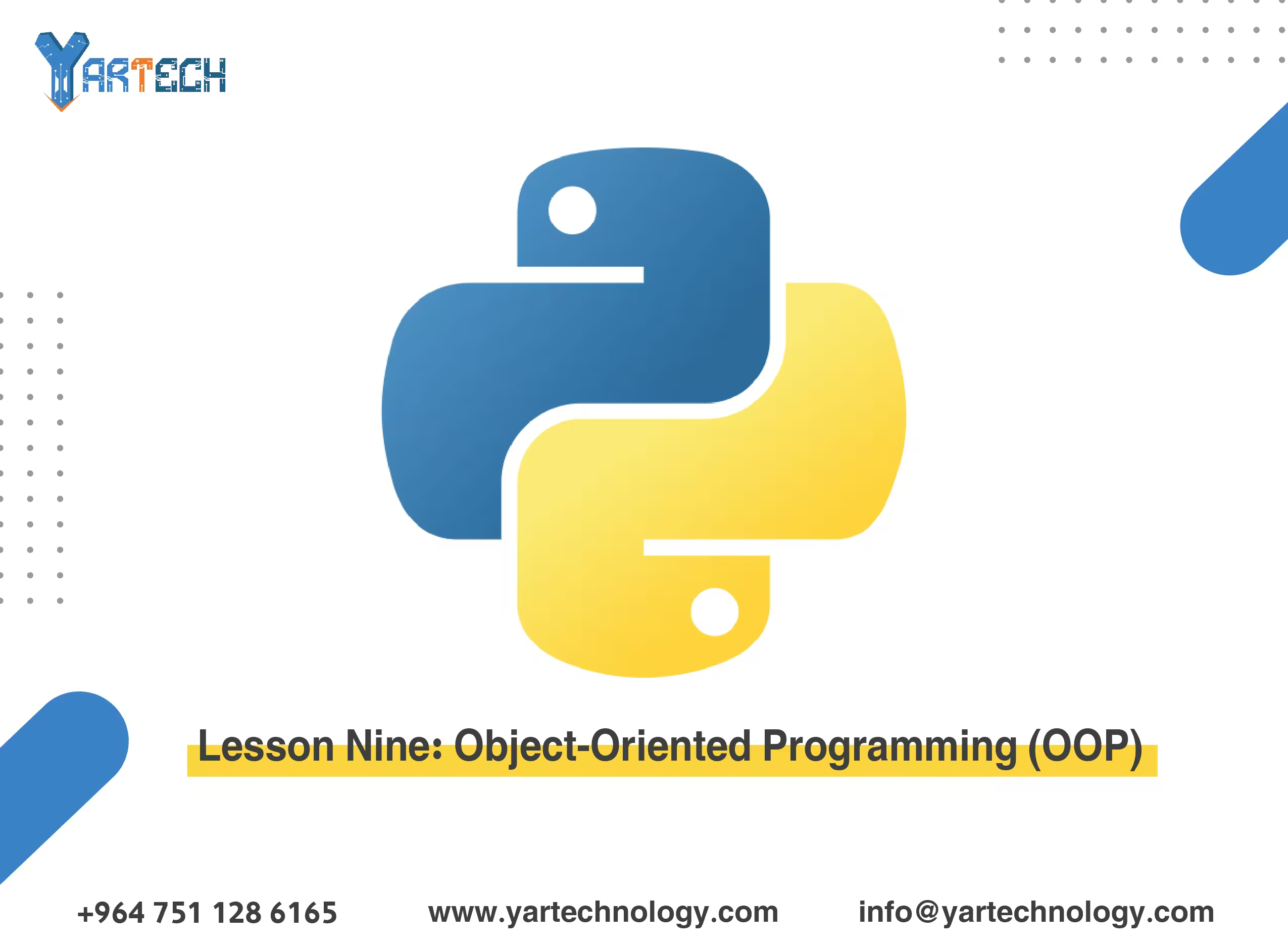
Lesson Nine: Object-Oriented Programming (OOP)
Welcome to the ninth lesson in our Python learning series! In this lesson, we will focus on Object-Oriented Programming (OOP) , a programming paradigm that relies on the use of classes and objects . OOP is one of the most common approaches in modern programming and is widely used in large applications such as game development, data analysis, and web development.
1. What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm based on the concept of objects , which represent real or virtual entities. Each object consists of:
- Attributes : Represent the data or state of the object.
- Methods : Represent the behaviors or actions that the object can perform.
Examples of Objects
-
Car :
- Attributes: Color, model, number of doors.
- Methods: Start the car, stop the car.
-
Person :
- Attributes: Name, age, city.
- Methods: Walk, talk.
2. Classes and Objects
A. Class
A class is a blueprint or template that defines how objects are constructed. You can think of a class as a "map" that specifies the attributes and methods that an object will contain.
B. Object
An object is an instance of a class. When you create an object, you are creating a new instance of the class with specific values.
3. Creating a Class and Object
A. Defining a Class
A class is defined using the class
keyword.
B. Creating an Object
To create an object from the class, you call the class and provide the required values.
4. The Constructor (__init__
) and the self
Keyword
A. The Constructor (__init__
)
The __init__
method is a special function used to initialize the object when it is created. It is automatically called when a new object is instantiated.
B. The self
Keyword
Every method inside a class needs a special parameter called self
. This parameter refers to the object itself and is used to access the object's attributes and methods.
5. Inheritance
Inheritance is a concept that allows you to create a new class based on an existing one. The new class is called the child class , and the original class is called the parent class .
A. Creating a Subclass
B. Using the Subclass
6. Encapsulation
Encapsulation is a concept that makes the attributes and methods inside an object inaccessible from outside directly. This is done using private attributes , which start with double underscores __
.
A. Using Private Attributes
B. Using the Object
7. Abstraction
Abstraction is a concept used to hide complex details and expose only the essential interfaces to the user. In Python, you can use abstract methods to achieve abstraction.
A. Creating an Abstract Class
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
B. Using the Object
circle = Circle(5)
print(circle.area()) # Output: 78.5
8. Practical Applications
Application 1: Student Management
Application 2: Banking System
9. Tips for Object-Oriented Programming
- Use Classes Appropriately : Only create classes if you need to represent objects with specific attributes and behaviors.
- Avoid Repetition Using Inheritance : If you have classes that share attributes or methods, use inheritance to reduce repetition.
- Encapsulation to Protect Data : Use private attributes to prevent direct access to an object's data from outside.
- Abstraction to Reduce Complexity : Use abstraction to hide complex details and make the code easier to use.
Conclusion
In this lesson, you learned the basics of Object-Oriented Programming (OOP) and how to use classes and objects in Python. OOP is a powerful tool that helps you organize your code and make it more reusable.
In the next lesson, we will discuss popular Python libraries like NumPy and Pandas and how to use them for data analysis. Keep practicing and don't hesitate to ask questions if you need help!
🚀 You're now on the right path to becoming a professional programmer!
Comments
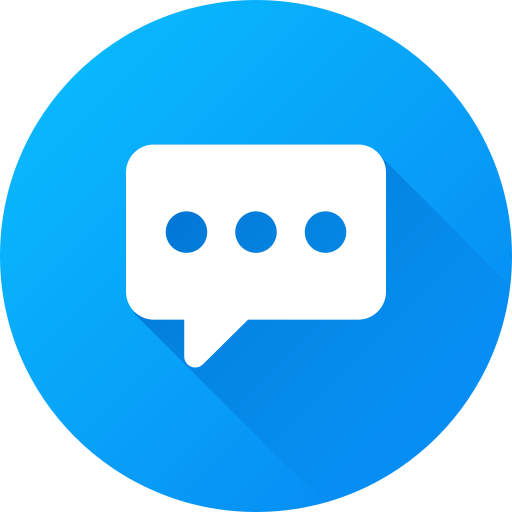
There are no comments
Please login to leave a review