Python File Handling
Lesson Five How to work with files in Python. How to read data from files and write data to files.
Learn Python
February 1, 2025
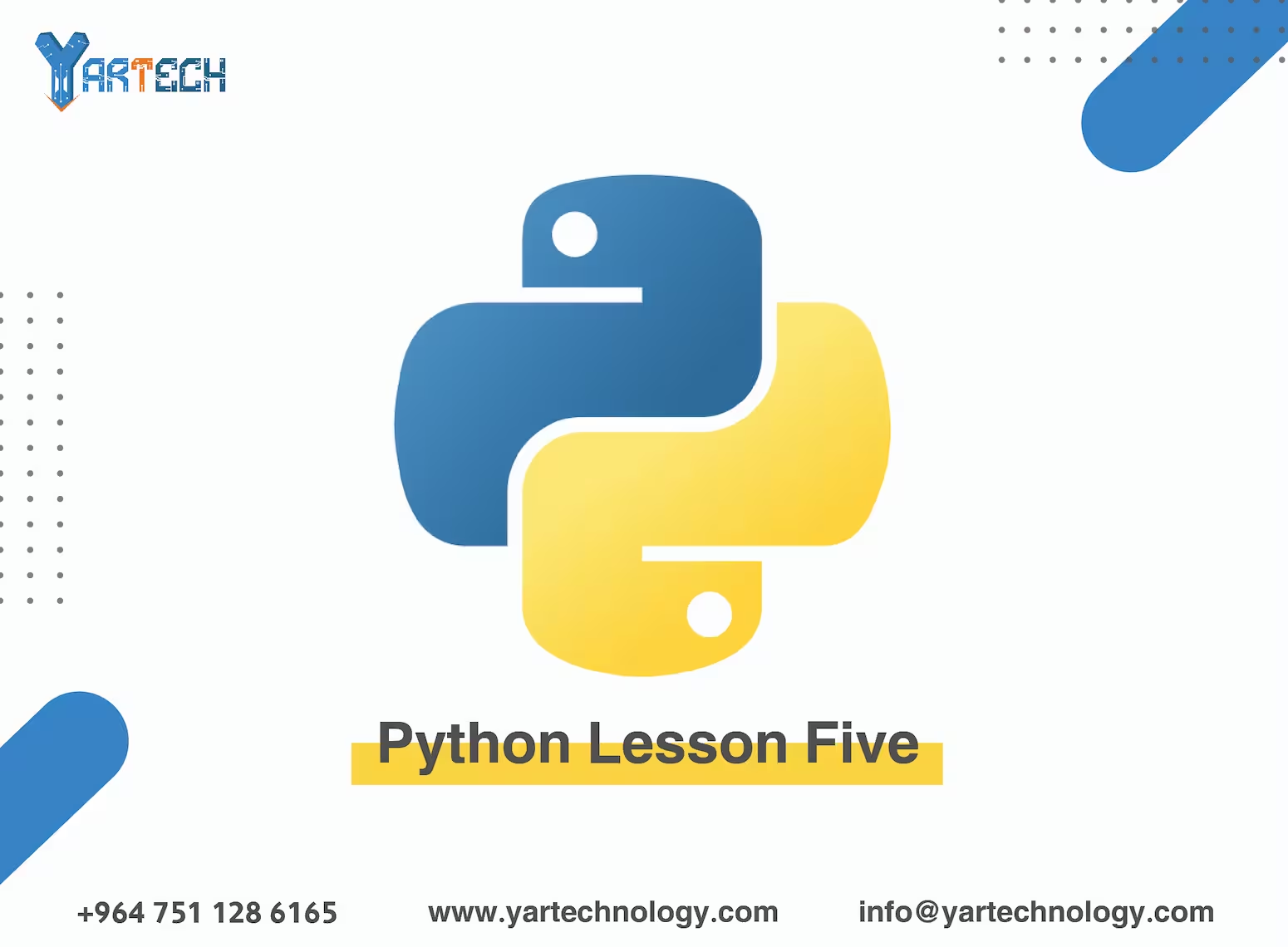
Lesson Five: File Handling
Welcome to the fifth lesson in our Python learning series! In this lesson, we will focus on how to handle files in Python. You will learn how to read data from files and write data to files. These skills are essential when working on projects that require storing data permanently or reading data from external files.
1. Opening Files
To work with files in Python, we use the open()
function. This function takes the file path and the mode (read, write, etc.) as its two main parameters.
A. File Modes
"r"
: Read mode. This is the default mode. If the file does not exist, an error will occur."w"
: Write mode. Creates the file if it doesn't exist, and if it does, it overwrites its contents."a"
: Append mode. Adds new data to the end of the file without deleting the existing content."x"
: Create mode. Creates the file only if it doesn't already exist. If the file exists, an error will occur.
# Open a file for reading
file = open("example.txt", "r")
# Open a file for writing
file = open("example.txt", "w")
B. Closing Files
After you finish working with a file, you should close it using the close()
method.
file = open("example.txt", "r")
# Some operations...
file.close()
Note : It's better to use the with
statement when handling files because it ensures the file is automatically closed even if an error occurs during execution.
with open("example.txt", "r") as file:
# Some operations...
pass # No need to manually close the file
2. Reading Data from Files
A. Reading the Entire Content
You can read the entire content of a file using the read()
method.
with open("example.txt", "r") as file:
content = file.read()
print(content)
B. Reading Lines One by One
If you want to read each line individually, you can use the readline()
method or iterate through the file using a for
loop.
# Read only the first line
with open("example.txt", "r") as file:
first_line = file.readline()
print(first_line)
# Read all lines
with open("example.txt", "r") as file:
for line in file:
print(line.strip()) # `strip()` removes whitespace and newline characters
C. Reading All Lines as a List
You can also read all lines in the file as a list using the readlines()
method.
with open("example.txt", "r") as file:
lines = file.readlines()
print(lines) # Output: A list containing each line as an element
3. Writing Data to Files
A. Writing to a New File
If you want to write new data to a file, you can use write mode "w"
.
with open("example.txt", "w") as file:
file.write("Hello, World!\n")
file.write("This is a new line.")
B. Appending Data to an Existing File
If you want to add new data to the end of an existing file without deleting the current content, use append mode "a"
.
with open("example.txt", "a") as file:
file.write("\nThis line was appended.")
4. Handling Binary Files
In addition to text files, you can also handle binary files such as images or compressed files. This is done using the modes "rb"
(reading binary files) and "wb"
(writing binary files).
A. Reading a Binary File
with open("image.jpg", "rb") as file:
binary_data = file.read()
B. Writing a Binary File
with open("new_image.jpg", "wb") as file:
file.write(binary_data)
5. Practical Applications
Application 1: Reading and Printing the Contents of a Text File
# Assuming we have a file named example.txt containing some text
with open("example.txt", "r") as file:
for line in file:
print(line.strip())
Application 2: Creating a File and Writing Data to It
# Create a new file and write some data
with open("data.txt", "w") as file:
file.write("Name: Ali\n")
file.write("Age: 25\n")
file.write("City: Riyadh\n")
# Read the file after writing
with open("data.txt", "r") as file:
print(file.read())
Application 3: Appending Data to an Existing File
# Add new data to an existing file
with open("data.txt", "a") as file:
file.write("Country: Saudi Arabia\n")
# Read the file after appending
with open("data.txt", "r") as file:
print(file.read())
6. Handling Exceptions
When working with files, you may encounter errors such as the file not existing or lacking permission to access it. Therefore, it's good practice to use exceptions (try-except
) to handle these cases.
try:
with open("nonexistent_file.txt", "r") as file:
content = file.read()
except FileNotFoundError:
print("The file does not exist!")
except PermissionError:
print("You do not have permission to access the file!")
7. Tips for File Handling
-
Use the
with
statement : Always usewith
when working with files because it ensures the file is automatically closed. -
Avoid frequent writes : When writing to a large file, try to minimize the number of times you write data to improve performance.
-
Check if the file exists : Before trying to read a file, make sure it exists using
os.path.exists()
.
import os
if os.path.exists("example.txt"):
with open("example.txt", "r") as file:
print(file.read())
else:
print("The file does not exist!")
Conclusion
In this lesson, you learned how to read data from files and write data to files using Python. These skills are very important when you need to store data permanently or read data from external files.
In the next lesson, we will discuss functions in more detail and how to organize your code using them. Keep practicing and don't hesitate to ask questions if you need help!
🚀 You're now on the right path to becoming a professional programmer!
Comments
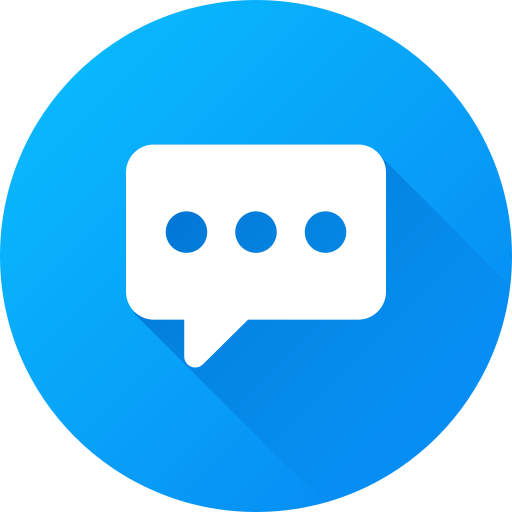
There are no comments
Please login to leave a review